Things Ian Says
Functional Programming in Javascript
Here is a collection of articles I’ve written around Functional Programming in Javascript. These should work well for you if you have some knowledge of Javascript and are interested in learning more about functional programming. However, it should also be useful for anyone who wants to understand common Functional Programming techniques, such as currying, partial evaluation and promises.
Memoization
Monday, 14 September 2015
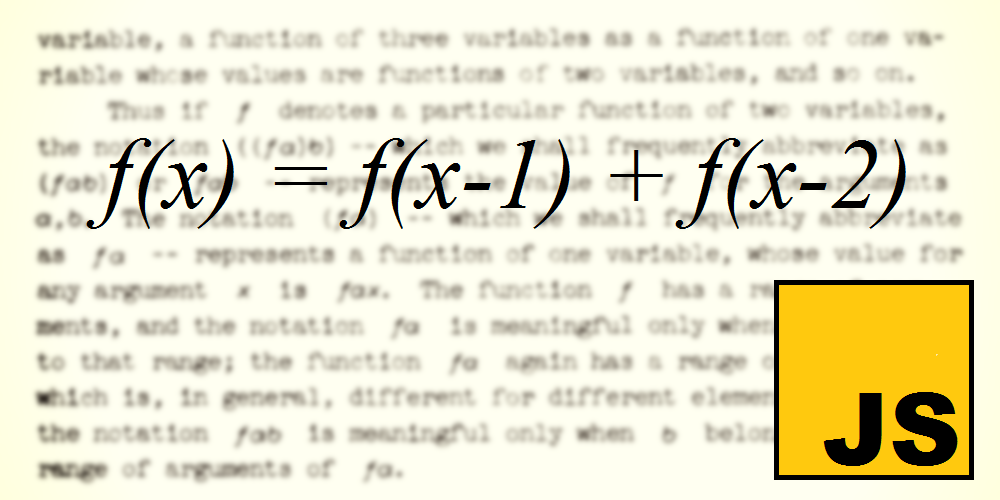
Some of my recent blog entries have dealt with using functional practices in Javascript. There are obviously downsides to functional programming as well, and one commonly stated one is around performance.
One area this applies to is in the large number of function calls resulting from this approach — particularly when we are writing recursive code.
We will give an example of this problem — calculating Fibonacci numbers — and then look at a technique known as memoization as a way of improving performance.
Functional Example
Saturday, 12 September 2015
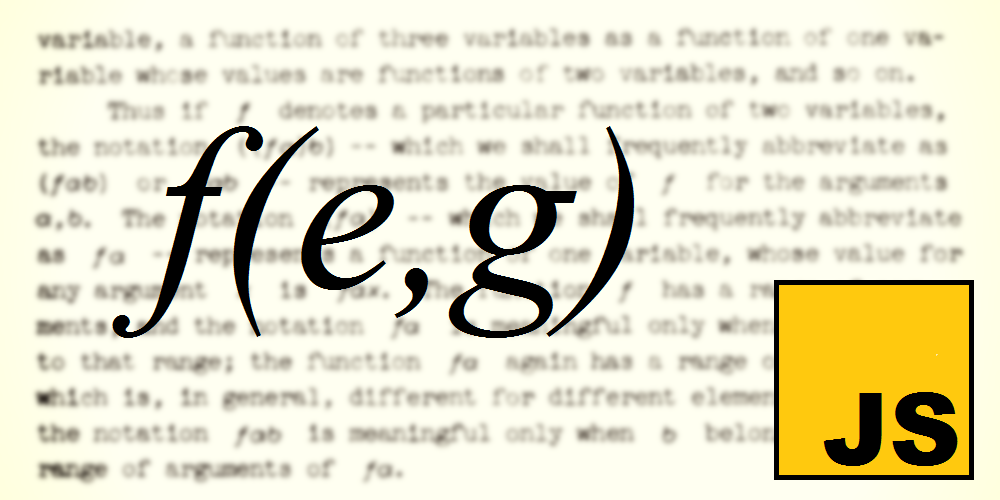
This blog entry will take the techniques discussed in the previous articles about functional Javascript and currying and apply them to an example problem.
Curried Javascript
Tuesday, 8 September 2015
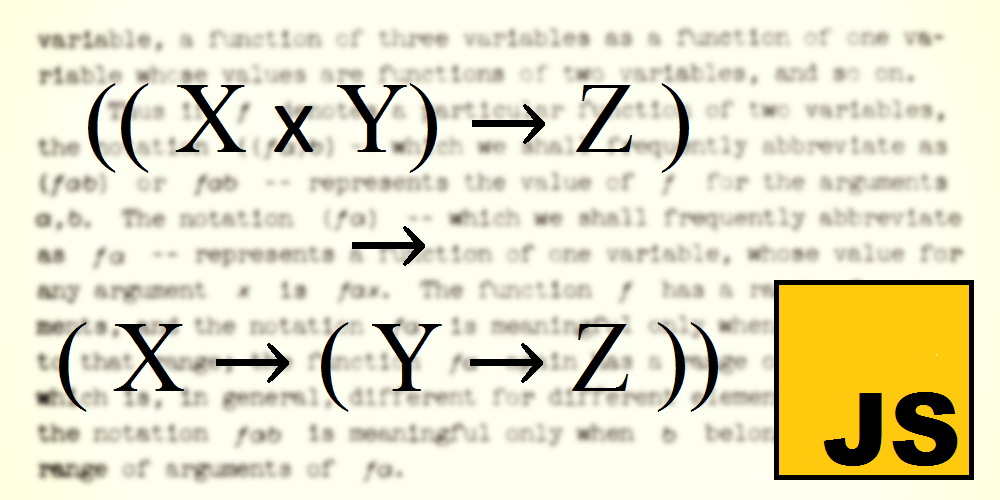
In my previous blog entry, we looked at how functions are first class entities in Javascript, what an anonymous (or lambda) function is, and how we create and use closures.
We will now look at another functional technique, known as currying, which builds on those concepts.
Functional Javascript
Thursday, 3 September 2015
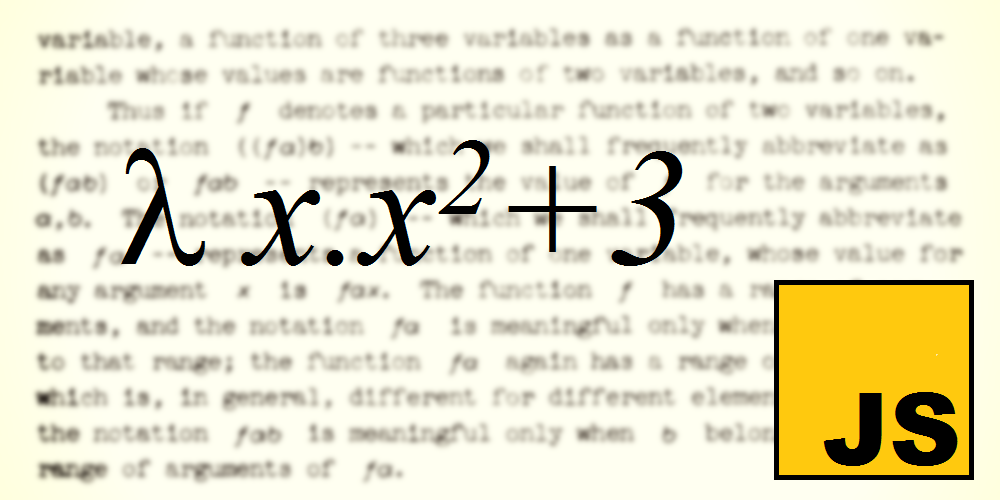
Functional programming is seeing an increase in popularity, as can be seen in rising adoption of languages like Scala, Clojure and Erlang. Even Java has introduced lambdas (more about lambdas, later).
Javascript supports a functional programming style and I find that working within that style makes many things simpler when developing Javascript. This is particularly true when working with promises.
This blog entry discusses some basic functional techniques and demonstrates how they can be used within Javascript. It should be useful to both people who wish to adopt a more functional style in their Javascript, and to people who just want to understand more about functional programming.
Promises in Javascript
Wednesday, 10 June 2015
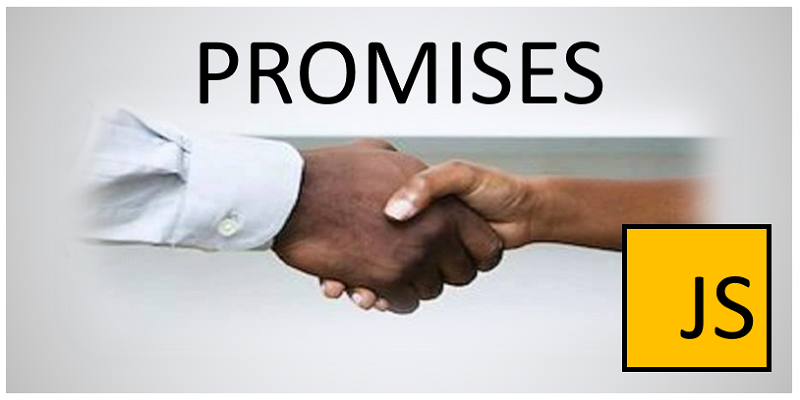
Javascript is single threaded and non-blocking, which means that lines of Javascript code are not necessarily executed in the same order they are written. This can cause problems with asynchronous behaviour, for example if the Javascript needs to call two different APIs in a specific order. This article discusses this challenge, and looks at the use of promises to address them.